クラス・構造体メニュー【構造体の作成・構造体の検索・構造体の整列・構造体の更新】
【STEP: 1】コード
reader.on('close', () => {
const N = Number(lines[0]);
class User {
constructor(n, o, b, s) {
this.n = n;
this.o = o;
this.b = b;
this.s = s;
}
info() {
console.log(`User{`);
console.log(`nickname : ${this.n}`);
console.log(`old : ${this.o}`);
console.log(`birth : ${this.b}`);
console.log(`state : ${this.s}`);
console.log(`}`);
}
}
for (let i = 1; i <= N; i++) {
const user = new User(...lines[i].split(' '));
user.info();
}
});
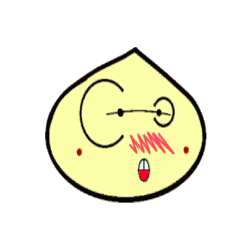
classの書式。
class クラス名 {
constructor(変数) {
this.クラス内で使用する変数 = 受け取った変数;
}
メソッド名() {
〇〇してね;
}
}
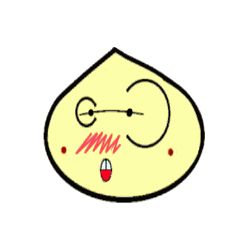
new User(...lines[i].split(' '))
でインスタンス生成。user.output()
で メソッドを呼び出し。
【STEP: 2】コード
reader.on('close', () => {
const N = Number(lines[0]);
const K = lines[N + 1];
class User { // クラス User を宣言して…
constructor(name, old, birth, state) { // コンストラクターで引数の受取り
this.name = name; // クラス内で使用する変数に受け取った値を代入
this.old = old; // 〃
this.birth = birth; // 〃
this.state = state; // 〃
}
info() { // メソッド(関数)
console.log(this.name);
}
}
for (let i = 1; i <= N; i++) {
user = new User(...lines[i].split(' ')); // インスタンス
if (user.old === K) { // user.old が K なら…
user.info(); // user.info() を呼び出して user.name を出力
}
}
});
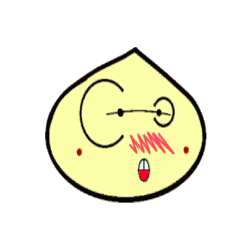
user.oldが K のときのみ user.info()
を呼び出しています。
【STEP: 3】コード
reader.on('close', () => {
const N = Number(lines[0]);
class User {
constructor(name, old, birth, state) {
this.name = name;
this.old = old;
this.birth = birth;
this.state = state;
}
info(){
console.log(this.name, this.old, this.birth, this.state);
}
}
const users = []; // User の インスタンスを保存するための配列
for (let i = 1; i <= N; i++) {
const [name, old, birth, state] = lines[i].split(' ');
users.push(new User(name, old, birth, state)); // インスタンス を生成して users に保存
}
users.sort((s, b) => s.old - b.old); // User の インスタンス を old を基準に並替え
users.forEach(user => {
user.info(); // User の メソッドを呼出
});
});
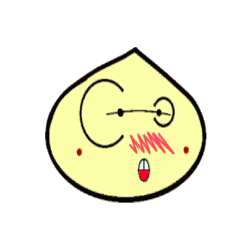
users は User の インスタンス を保存するための配列。old を基準にインスタンスの並べ替えを行っています。
【FINAL】コード
reader.on('close', () => {
class User { // クラス User を宣言して…
constructor(nickname, old, birth, state) { // コンストラクターで引数の受取り
this.name = nickname; // クラス内の変数に受け取った値を代入
this.old = old; // 〃
this.birth = birth; // 〃
this.state = state; // 〃
}
changeName(new_name) { // メソッド(関数)
this.name = new_name; // インスタンスの変数を上書き
}
info() { // メソッド(関数)
console.log(this.kname, this.old, this.birth, this.state); // 出力
}
}
const users = []; // User の インスタンスを保存するための配列
const [N, K] = lines[0].split(' ').map(Number);
for (let i = 1; i <= N; i++) {
const [name, old, birth, state] = lines[i].split(' ');
users.push(new User(name, old, birth, state)); // インスタンス を生成して保存
}
for (let i = N + 1; i <= N + K; i++) {
const [A, M] = lines[i].split(' ');
users[A - 1].changeName(M); // インスタンス.changeName(M) でメソッドを呼び出し
}
users.forEach(user => {
user.info();
});
});
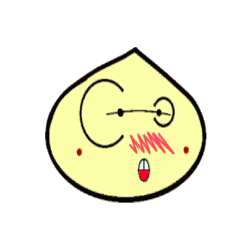
クラス内に メソッド changeName(name)
を設置し 配列 users の 添字 が [A-1] であれば 呼び出して インスタンスの 値を更新しています。
コメント